Docker has revolutionized how we develop, deploy, and run applications. This powerful containerization platform offers a consistent environment across development, testing, and production stages, eliminating the infamous "it works on my machine" problem. In this comprehensive guide, we'll explore Docker fundamentals through a practical lens.
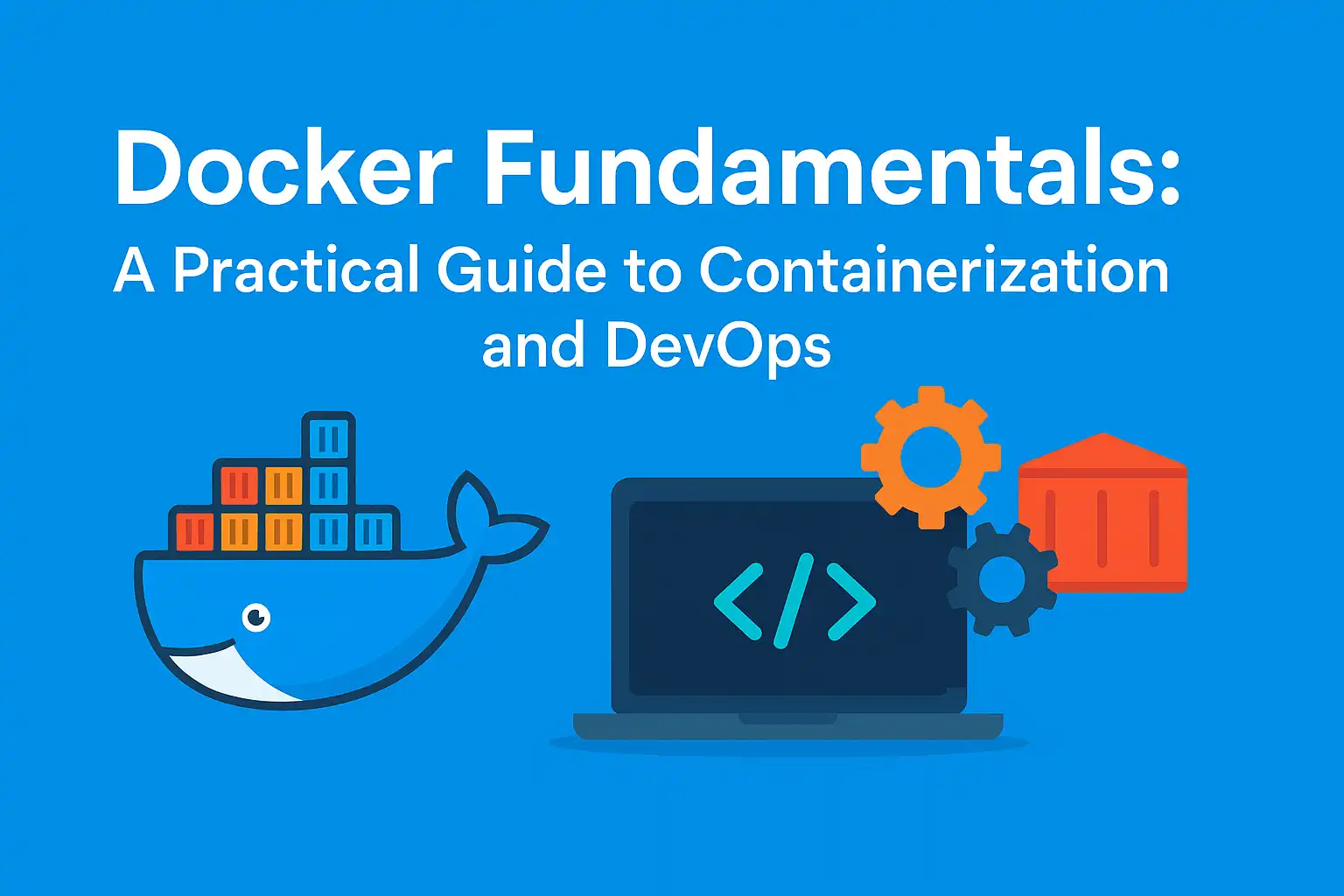
Table of Contents #
- What is Docker?
- The "Why" Behind Docker
- Key Benefits of Docker
- What Problems Does Docker Solve?
- What Problems Remain Outside Docker's Scope?
- Getting Started with Docker
- Introducing Our Docker Practical Series
- Conclusion
What is Docker? #
Docker is an open platform for developing, shipping, and running applications in containers. Containers package an application with all its dependencies into a standardized unit, ensuring that it works seamlessly in any environment where Docker is installed.
Unlike virtual machines that virtualize an entire operating system, Docker containers share the host system's kernel and isolate the application processes from the rest of the system. This makes them significantly more lightweight and efficient.
┌────────────────────────────────────────────────────────────┐
│ │
│ DOCKER │
│ │
│ ┌───────────────────────────────────────────────────────┐ │
│ │ │ │
│ │ Containers: Lightweight, portable units that │ │
│ │ package applications and dependencies │ │
│ │ │ │
│ └───────────────────────────────────────────────────────┘ │
│ │
│ ┌───────────────────────────────────────────────────────┐ │
│ │ │ │
│ │ Images: Read-only templates for creating containers │ │
│ │ │ │
│ └───────────────────────────────────────────────────────┘ │
│ │
│ ┌───────────────────────────────────────────────────────┐ │
│ │ │ │
│ │ Docker Engine: Technology that runs containers │ │
│ │ │ │
│ └───────────────────────────────────────────────────────┘ │
│ │
└────────────────────────────────────────────────────────────┘
The "Why" Behind Docker #
Traditional software deployment faces numerous challenges:
┌─────────────────────────────┐ ┌─────────────────────────────┐
│ Development Environment │ │ Production Environment │
│ │ │ │
│ ┌─────────┐ ┌─────────┐ │ │ ┌─────────┐ ┌─────────┐ │
│ │ App A │ │ App B │ │ │ │ App A │ │ App B │ │
│ │ v1.2 │ │ v2.0 │ │ │ │ v1.0 │ │ v1.8 │ │
│ └─────────┘ └─────────┘ │ │ └─────────┘ └─────────┘ │
│ │ │ │
│ ┌─────────┐ ┌─────────┐ │ │ ┌─────────┐ ┌─────────┐ │
│ │ Lib X │ │ Lib Y │ │ │ │ Lib X │ │ Lib Y │ │
│ │ v2.2 │ │ v3.0 │ │ │ │ v1.5 │ │ v2.1 │ │
│ └─────────┘ └─────────┘ │ │ └─────────┘ └─────────┘ │
│ │ │ │
│ ┌─────────────────────┐ │ │ ┌─────────────────────┐ │
│ │ OS (macOS/Windows) │ │ │ │ OS (Linux) │ │
│ └─────────────────────┘ │ │ └─────────────────────┘ │
└─────────────────────────────┘ └─────────────────────────────┘
│ │
│ Deployment │
└─────────────────────────────────┘
"Works on my machine!"
Before Docker, developers regularly faced the infamous "it works on my machine" problem due to differences between development, testing, and production environments. Docker emerged to solve these inconsistencies by containerizing applications.
Key Benefits of Docker #
Docker offers numerous advantages that have made it an essential tool in modern development:
- Consistency: Identical environment from development to production
- Portability: Run anywhere Docker is installed
- Isolation: Applications run independently with their dependencies
- Efficiency: Lightweight compared to traditional VMs
- Scalability: Easy to scale applications horizontally
- Version Control: Images are versioned for easy rollback
- Rapid Deployment: Fast application startup times
- Resource Management: CPU and memory constraints
- Microservices Architecture: Perfect fit for microservices
┌─────────────────────────────────────────────────────────────┐
│ Docker Benefits │
│ │
│ ┌───────────┐ ┌───────────┐ ┌────────────┐ │
│ │Consistency│ │Portability│ │ Isolation │ │
│ └───────────┘ └───────────┘ └────────────┘ │
│ │
│ ┌───────────┐ ┌───────────┐ ┌────────────┐ │
│ │Efficiency │ │Scalability│ │ Version │ │
│ │ │ │ │ │ Control │ │
│ └───────────┘ └───────────┘ └────────────┘ │
│ │
│ ┌───────────┐ ┌───────────┐ ┌────────────┐ │
│ │ Rapid │ │ Resource │ │Microservice│ │
│ │Deployment │ │Management │ │ Support │ │
│ └───────────┘ └───────────┘ └────────────┘ │
│ │
└─────────────────────────────────────────────────────────────┘
What Problems Does Docker Solve? #
Docker addresses several critical challenges in modern application development:
- Environment Consistency: Eliminates "works on my machine" syndrome
- Dependency Isolation: Prevents conflicts between applications
- Resource Efficiency: Uses less resources than virtual machines
- Deployment Speed: Containers start in seconds instead of minutes
- Application Portability: Run anywhere Docker is installed
- Version Management: Easy rollbacks and updates
- DevOps Integration: Streamlines CI/CD pipelines
What Problems Remain Outside Docker's Scope? #
Despite its advantages, Docker has limitations:
- Stateful Applications: Containers are ephemeral by design, making stateful applications challenging
- GUI Applications: Not designed for graphical applications
- Cross-Container Networking: Can be complex to set up
- Security Concerns: Containers share the host kernel, potential security risks
- Persistent Storage: Requires careful planning
- Windows Application Support: Limited compared to Linux applications
- Learning Curve: Requires new skills and organizational changes
- Container Orchestration: Docker alone doesn't handle complex orchestration (solved by Kubernetes)
- Monitoring: Requires additional tools for production monitoring
Getting Started with Docker #
To get started with Docker, you'll need to:
- Install Docker: Docker Desktop for macOS and Windows, or Docker Engine for Linux
- Understand basic concepts: Images, containers, volumes, and networks
- Learn essential commands:
docker run
,docker build
,docker pull
, etc. - Create your first Dockerfile: Define how your application should be containerized
Docker can be installed on various operating systems:
For macOS and Windows #
- Download Docker Desktop
- Follow the installation wizard
- Launch Docker Desktop
For Linux (Ubuntu) #
# Update package index
sudo apt-get update
# Install prerequisites
sudo apt-get install \
apt-transport-https \
ca-certificates \
curl \
gnupg \
lsb-release
# Add Docker's official GPG key
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo gpg --dearmor -o /usr/share/keyrings/docker-archive-keyring.gpg
# Set up the stable repository
echo \
"deb [arch=amd64 signed-by=/usr/share/keyrings/docker-archive-keyring.gpg] https://download.docker.com/linux/ubuntu \
$(lsb_release -cs) stable" | sudo tee /etc/apt/sources.list.d/docker.list > /dev/null
# Install Docker Engine
sudo apt-get update
sudo apt-get install docker-ce docker-ce-cli containerd.io
# Add your user to the docker group (to run docker without sudo)
sudo usermod -aG docker $USER
Introducing Our Docker Practical Series #
This article is the first in our comprehensive Docker Practical Guide series. Throughout this series, we'll explore Docker's features and capabilities through hands-on examples and practical labs.
Our upcoming articles will cover:
- Docker Architecture and Ecosystem: A Developer's Guide | Docker Practical Series #2
- Docker Cheat Sheet: The Ultimate Quick Reference Guide | Docker Practical Series #3
- Containerizing a FastAPI Application with Docker: A Step-by-Step Guide | Docker Practical Series #4
- Docker Volumes & Data Persistence: The Complete Guide for Reliable Container Storage | Docker Practical Series #5
- Mastering Docker Networking: A Comprehensive Guide to Container Communication | Docker Practical Series #6
- Optimizing Docker Images with Multi-Stage Builds: Reduce Size & Boost Security | Docker Practical Series #7
- Docker Image Management Best Practices: Optimize, Secure & Automate | Docker Practical Series #8
- Mastering Docker Swarm: Simplified Container Orchestration for Scalable Microservices | Docker Practical Series #9
- Advanced Docker Build Techniques with Bake and BuildKit: Optimize Your Container Workflows | Docker Practical Series #10
- Local Cloud Development with Docker and LocalStack: Emulate AWS Services Locally | Docker Practical Series #11
Each article will provide practical examples and step-by-step instructions to help you master Docker concepts through real-world applications.
Conclusion #
Docker has revolutionized application development and deployment by solving key challenges in software delivery. Understanding Docker fundamentals is essential for any modern developer or DevOps professional.
In this series, we'll move beyond theory and dive into practical applications of Docker with hands-on examples. Whether you're just getting started with containerization or looking to optimize your Docker workflows, our practical guide will provide valuable insights and techniques.
Join us as we explore the world of Docker through a practical lens, starting with the basics and progressing to advanced concepts. By the end of this series, you'll have a solid foundation in Docker and be ready to implement containerization strategies in your own projects.
What challenges are you facing with your deployment process that Docker might solve? Share your thoughts in the comments below!