Docker's revolutionary impact on software development is built upon a robust and well-designed architecture. Understanding this architecture is crucial for effectively utilizing Docker in your development workflow. In this article, we'll break down Docker's components and explain how they work together to create a powerful containerization platform.
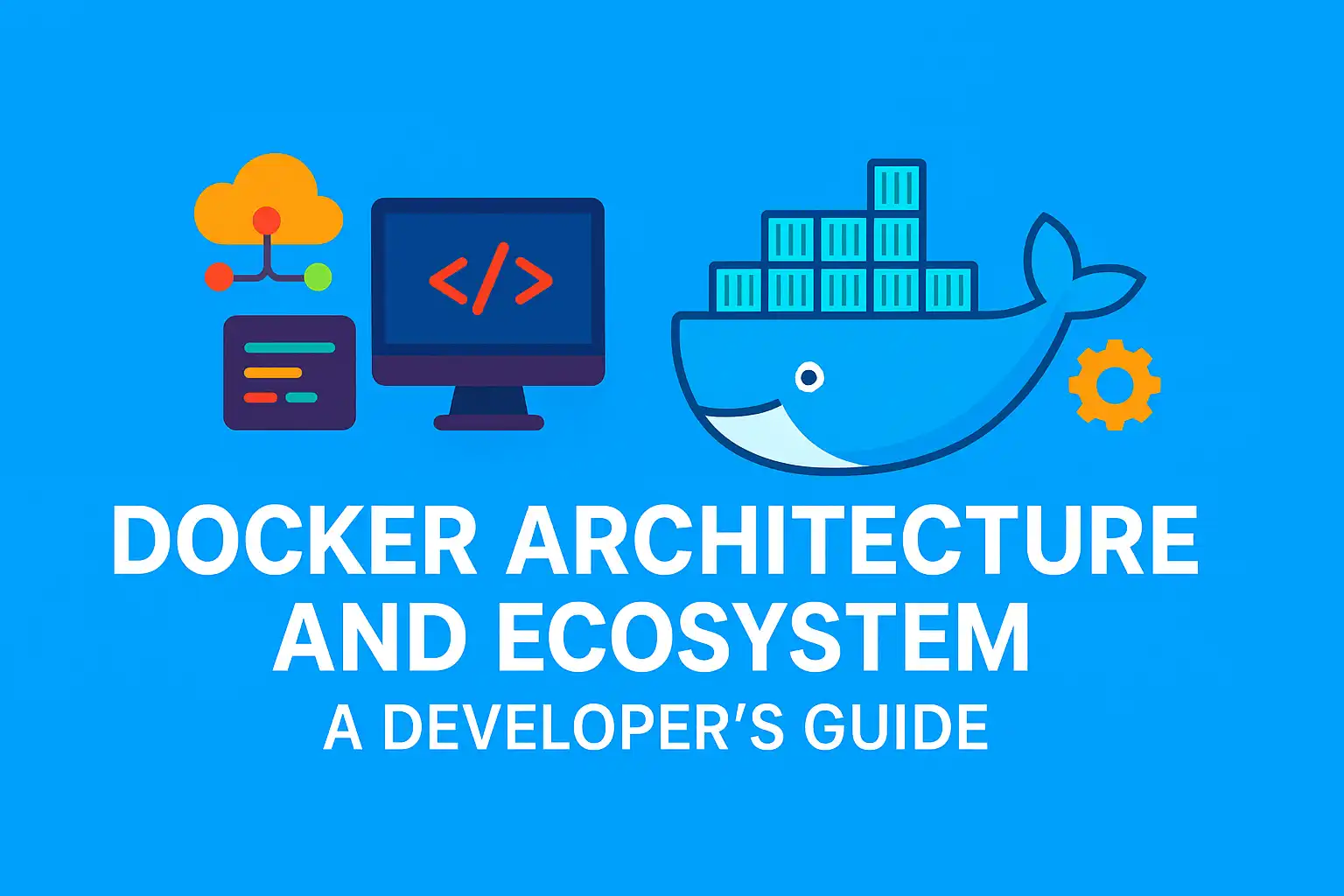
Table of Contents #
- Docker's Client-Server Architecture
- Docker Engine: The Core of Docker
- Docker Objects
- Docker Registry and Docker Hub
- Docker Command Structure
- Docker vs. Virtual Machines: Architecture Differences
- The Extended Docker Ecosystem
- Docker's Role in Modern DevOps
- Conclusion
Docker's Client-Server Architecture #
Docker uses a client-server architecture with several key components working together:
┌────────────────────────────────────────────────────────────┐
│ │
│ Docker Architecture │
│ │
│ ┌─────────────┐ ┌───────────────────────────┐ │
│ │ │ REST API │ │ │
│ │ Docker CLI ├──────────► Docker Daemon │ │
│ │ │ │ │ │
│ └─────────────┘ └────────────┬──────────────┘ │
│ │ │
│ │ Manages │
│ ▼ │
│ ┌─────────────┐ ┌────────────────────────────┐ │
│ │ │ Pull/ │ │ │
│ │ Registry ◄──────────┤ Images & Containers │ │
│ │ │ Push │ │ │
│ └─────────────┘ └────────────────────────────┘ │
│ │
└────────────────────────────────────────────────────────────┘
The architecture consists of:
- Docker Client: The primary way users interact with Docker through commands
- Docker Daemon (dockerd): A background service that manages Docker objects and responds to requests from the Docker client
- REST API: The interface that programs use to communicate with the Docker daemon
- Registry: A repository for Docker images (like Docker Hub)
This client-server design allows for flexibility: you can run the Docker client on your local machine while connecting to a remote Docker daemon running on a different system.
Docker Engine: The Core of Docker #
Docker Engine is the core technology that powers Docker. It's a client-server application with these main components:
┌────────────────────────────────────────────────────────────┐
│ Docker Engine │
│ │
│ ┌────────────────────────────────────────────────────┐ │
│ │ │ │
│ │ Docker Daemon (dockerd) │ │
│ │ - Manages Docker objects │ │
│ │ - Listens for API requests │ │
│ │ - Handles container lifecycle │ │
│ │ │ │
│ └────────────────────────────────────────────────────┘ │
│ ▲ │
│ │ │
│ │ REST API │
│ │ │
│ ▼ │
│ ┌────────────────────────────────────────────────────┐ │
│ │ │ │
│ │ Docker CLI (docker) │ │
│ │ - Command-line interface │ │
│ │ - Interacts with the daemon via API │ │
│ │ │ │
│ └────────────────────────────────────────────────────┘ │
│ │
└────────────────────────────────────────────────────────────┘
Docker Daemon (dockerd) #
The Docker daemon is a background service that:
- Listens for Docker API requests
- Manages Docker objects such as images, containers, networks, and volumes
- Communicates with other daemons to manage Docker services
In production environments, the daemon typically runs as a system service:
# Check the status of the Docker daemon
systemctl status docker
# View Docker daemon logs
journalctl -u docker.service
# Configure daemon parameters
sudo nano /etc/docker/daemon.json
Docker CLI #
The command-line interface for interacting with the Docker daemon. Most Docker users primarily work with the CLI, which simplifies complex operations into simple commands like:
# Run a container
docker run nginx
# Build an image
docker build -t myapp .
# List running containers
docker ps
REST API #
The Docker API is RESTful, allowing other programs and tools to interact with the Docker daemon. This is what enables tools like Docker Desktop, Portainer, and various CI/CD systems to manage Docker resources programmatically.
Developers can use this API to:
- Create custom Docker management tools
- Integrate Docker with other systems
- Automate container operations
Docker Objects #
Docker objects are the building blocks of your Docker applications:
Images #
Images are read-only templates that contain:
- The application code
- Libraries and dependencies
- System tools
- Other files needed to run an application
They're built from the instructions in a Dockerfile and serve as the basis for containers.
# List images
docker images
# Pull an image
docker pull nginx:latest
# Build an image
docker build -t myapp:1.0 .
Containers #
Containers are runnable instances of images. They encapsulate:
- The application
- Its dependencies
- A filesystem
- Environment variables
- Network interfaces
Containers are isolated from each other and the host system, yet efficient because they share the host's kernel.
# Run a container
docker run -d -p 80:80 nginx
# List running containers
docker ps
# Stop a container
docker stop container_id
Volumes #
Volumes provide persistent storage for containers:
- Managed by Docker
- Independent of container lifecycle
- Can be shared between containers
# Create a volume
docker volume create my_data
# Run a container with a volume
docker run -v my_data:/app/data nginx
Networks #
Networks enable communication between containers and with the outside world:
- Bridge networks (default)
- Host networks
- Overlay networks
- Macvlan networks
# Create a network
docker network create my_network
# Run a container on a specific network
docker run --network=my_network nginx
Docker Registry and Docker Hub #
A Docker registry is a storage and distribution system for Docker images.
┌─────────────────────────────────────────────────────────────┐
│ Docker Registry Workflow │
│ │
│ ┌────────────┐ Push ┌─────────────────────┐ │
│ │ │─────────►│ │ │
│ │ Developers│ │ Docker Registry │ │
│ │ │◄─────────│ (Public/Private) │ │
│ └────────────┘ Pull │ │ │
│ ▲ └─────────────────────┘ │
│ │ ▲ │
│ │ Pull │ Push │
│ │ │ │
│ ▼ ▼ │
│ ┌────────────┐ ┌─────────────────────┐ │
│ │ │ │ │ │
│ │ Production │ │ CI/CD Systems │ │
│ │ │ │ │ │
│ └────────────┘ └─────────────────────┘ │
│ │
└─────────────────────────────────────────────────────────────┘
Docker Hub #
Docker Hub is the default public registry for Docker images. It provides:
- Official images maintained by Docker and software vendors
- Community images shared by developers
- Private repositories for your own images
- Automated builds from GitHub or BitBucket repositories
# Pull an image from Docker Hub
docker pull python:3.10
# Push an image to Docker Hub
docker push username/myapp:latest
# Search for images
docker search postgresql
Private Registries #
Many organizations use private registries for proprietary images:
- Amazon Elastic Container Registry (ECR)
- Google Container Registry (GCR)
- Azure Container Registry (ACR)
- Self-hosted options like Harbor or GitLab Container Registry
# Log in to a private registry
docker login registry.example.com
# Pull from a private registry
docker pull registry.example.com/team/image:tag
# Push to a private registry
docker push registry.example.com/team/image:tag
Docker Command Structure #
Docker CLI commands follow a consistent structure:
docker [OPTIONS] COMMAND [ARGUMENTS]
┌─────────────────────────────────────────────────────────────┐
│ Docker Command Structure │
│ │
│ ┌────────────┐ ┌────────────┐ ┌────────────────────┐ │
│ │ │ │ │ │ │ │
│ │ docker │─► │ COMMAND │─► │ ARGUMENTS │ │
│ │ │ │ │ │ │ │
│ └────────────┘ └────────────┘ └────────────────────┘ │
│ │
│ Examples: │
│ docker run -p 8080:80 nginx │
│ docker build -t myapp:latest . │
│ docker volume create mydata │
│ │
└─────────────────────────────────────────────────────────────┘
Commands are organized into logical groups:
- Container Management:
run
,start
,stop
,ps
,exec
- Image Management:
build
,pull
,push
,images
,rmi
- Volume Management:
volume create
,volume ls
,volume rm
- Network Management:
network create
,network ls
,network rm
- System Commands:
info
,version
,system prune
- Compose and Swarm:
compose
,swarm
,service
,stack
Docker vs. Virtual Machines: Architecture Differences #
Docker containers differ fundamentally from virtual machines in their architecture:
┌───────────────────────────────┐ ┌───────────────────────────────┐
│ Virtual Machines │ │ Docker │
│ │ │ │
│ ┌─────────┐ ┌─────────┐ │ │ ┌─────────┐ ┌─────────┐ │
│ │ App A │ │ App B │ │ │ │ App A │ │ App B │ │
│ └─────────┘ └─────────┘ │ │ └─────────┘ └─────────┘ │
│ ┌─────────┐ ┌─────────┐ │ │ ┌─────────┐ ┌─────────┐ │
│ │ Bins/ │ │ Bins/ │ │ │ │ Bins/ │ │ Bins/ │ │
│ │ Libs │ │ Libs │ │ │ │ Libs │ │ Libs │ │
│ └─────────┘ └─────────┘ │ │ └─────────┘ └─────────┘ │
│ ┌─────────┐ ┌─────────┐ │ │ │
│ │ Guest │ │ Guest │ │ │ Docker Engine │
│ │ OS │ │ OS │ │ │ │
│ └─────────┘ └─────────┘ │ │ │
│ │ │ │
│ Hypervisor │ │ Host OS │
│ │ │ │
│ Host OS │ │ │
│ │ │ │
│ Hardware │ │ Hardware │
└───────────────────────────────┘ └───────────────────────────────┘
Key differences:
- Resource Efficiency: Containers share the host OS kernel, making them much lighter and faster than VMs
- Startup Time: Containers start in seconds vs. minutes for VMs
- Isolation Level: VMs provide stronger isolation but with higher overhead
- Resource Overhead: Containers use fewer resources, allowing higher density
- OS Compatibility: Containers must be compatible with the host OS kernel
The Extended Docker Ecosystem #
Docker's ecosystem extends beyond the core components to include several tools that enhance its functionality:
Docker Compose #
A tool for defining and running multi-container Docker applications using YAML files:
version: "3"
services:
web:
image: nginx
ports:
- "80:80"
db:
image: postgres
volumes:
- db_data:/var/lib/postgresql/data
volumes:
db_data:
This makes complex, multi-container setups more manageable.
Docker Swarm #
Docker's native clustering and orchestration solution that turns a group of Docker hosts into a single virtual Docker host. It enables:
- Service deployment across multiple nodes
- Load balancing
- Service discovery
- Rolling updates
Docker BuildKit #
An improved build system that offers:
- Faster builds with better caching
- Concurrent build stages
- Build secrets
- Multi-platform builds
Docker Extensions #
Docker Desktop extensions that add new functionality to your Docker environment, such as:
- Development environments
- Database management
- Kubernetes integration
- Network analysis
Docker's Role in Modern DevOps #
Docker sits at the heart of modern DevOps practices:
Continuous Integration/Continuous Deployment (CI/CD):
- Consistent build environments
- Reproducible testing
- Simplified deployment pipelines
Microservices Architecture:
- Natural fit for containerized services
- Independent scaling and deployment
- Technology diversity
Infrastructure as Code:
- Defining container configurations as code
- Version-controlled infrastructure
- Reproducible environments
Cloud-Native Development:
- Seamless deployment to cloud platforms
- Consistent local and cloud environments
- Integration with cloud services
Conclusion #
Understanding Docker's architecture is essential for effectively leveraging this powerful containerization platform. The client-server model, coupled with Docker's various components like images, containers, volumes, and networks, provides a flexible and efficient system for developing, deploying, and running applications.
Docker's architecture and ecosystem continue to evolve, with ongoing improvements to performance, security, and developer experience. As containerization becomes increasingly central to modern software development, a deep understanding of Docker's architecture will serve as a valuable foundation for your development and DevOps practices.
In the next article in our Docker Practical Guide series, we'll dive into practical containerization with Docker, exploring how to containerize a real-world application using the FastAPI framework. Stay tuned for hands-on examples and step-by-step instructions to put these architectural concepts into practice.
What aspects of Docker's architecture or ecosystem are you most interested in exploring further? Let us know in the comments!